try-catch
is a standard way of handling exceptions that is almost ubiquitous in modern programming languages. Besides, it's added to solidity
0.6.
In this chapter, we will introduce how to use try-catch
to handle exceptions in smart contracts.
try-catch
In solidity
, try-catch
can only be used for external
function or call constructor
(considered external
function) when creating contracts. The basic syntax is as follows:
externalContract.f()
is a function call of an external contract, try
module runs if the call succeeds, while catch
module runs if the call fails.
You can also use this.f()
instead of externalContract.f()
. this.f()
is also considered as an external call, but can't be used in the constructor because the contract has not been created at that time.
If the called function has a return value, then returns(returnType val)
must be declared after try
, and the returned variable can be used in try
module. In the case of contract creation, the returned value is a newly created contract variable.
Besides, catch
module supports catching special exception causes:
try-catch
actual combat
OnlyEven
We create an external contract OnlyEven
and use try-catch
to handle exceptions:
OnlyEven
contract contains a constructor and an onlyEven
function.
- constructor has one argument
a
, whena=0
,require
will throw an exception; Whena=1
,assert
will throw an exception. All other conditions are normal. onlyEven
function has one argumentb
, whenb
is odd,require
will throw an exception.
Handle external function call exceptions
First, define some events and state variables in TryCatch
contract:
SuccessEvent
is the event that will be released when the call succeeds, while CatchEvent
and CatchByte
are the events that will be released when an exception is thrown, corresponding to require/revert
and assert
exceptions respectively. even
is a state variable of OnlyEven
contract type.
Then we use try-catch
in execute
function to handle exceptions in the call to the external function onlyEven
:
verify on remix
When running execute(0)
, because 0
is even, satisfy require(b % 2 == 0, "Ups! Reverting");
, so no exception is thrown. The call succeeds and SuccessEvent
is released.
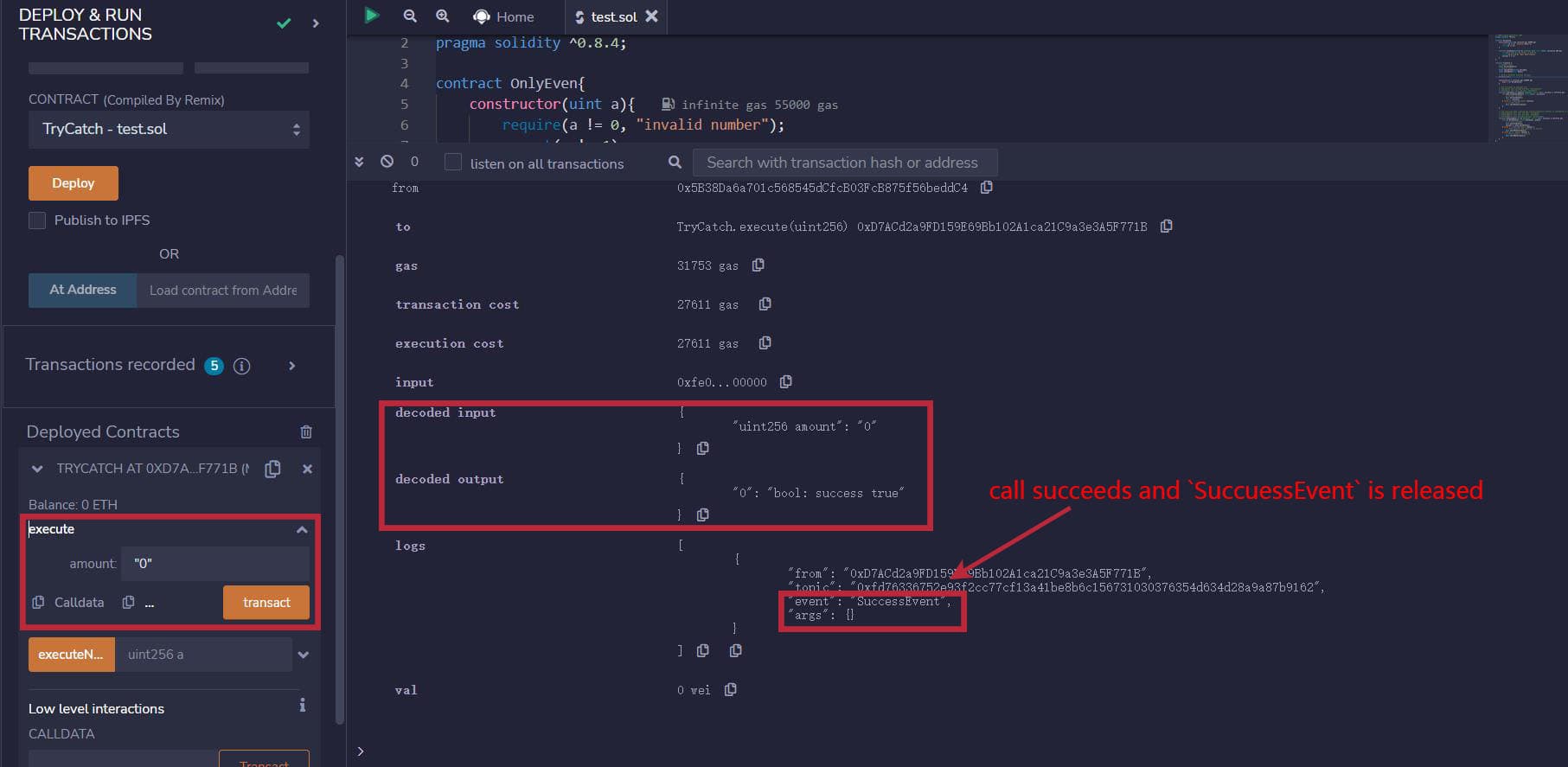
When running execute(1)
, because 1
is odd, doesn't satisfy require(b % 2 == 0, "Ups! Reverting");
, so the exception is thrown. The call fails and CatchEvent
is released.
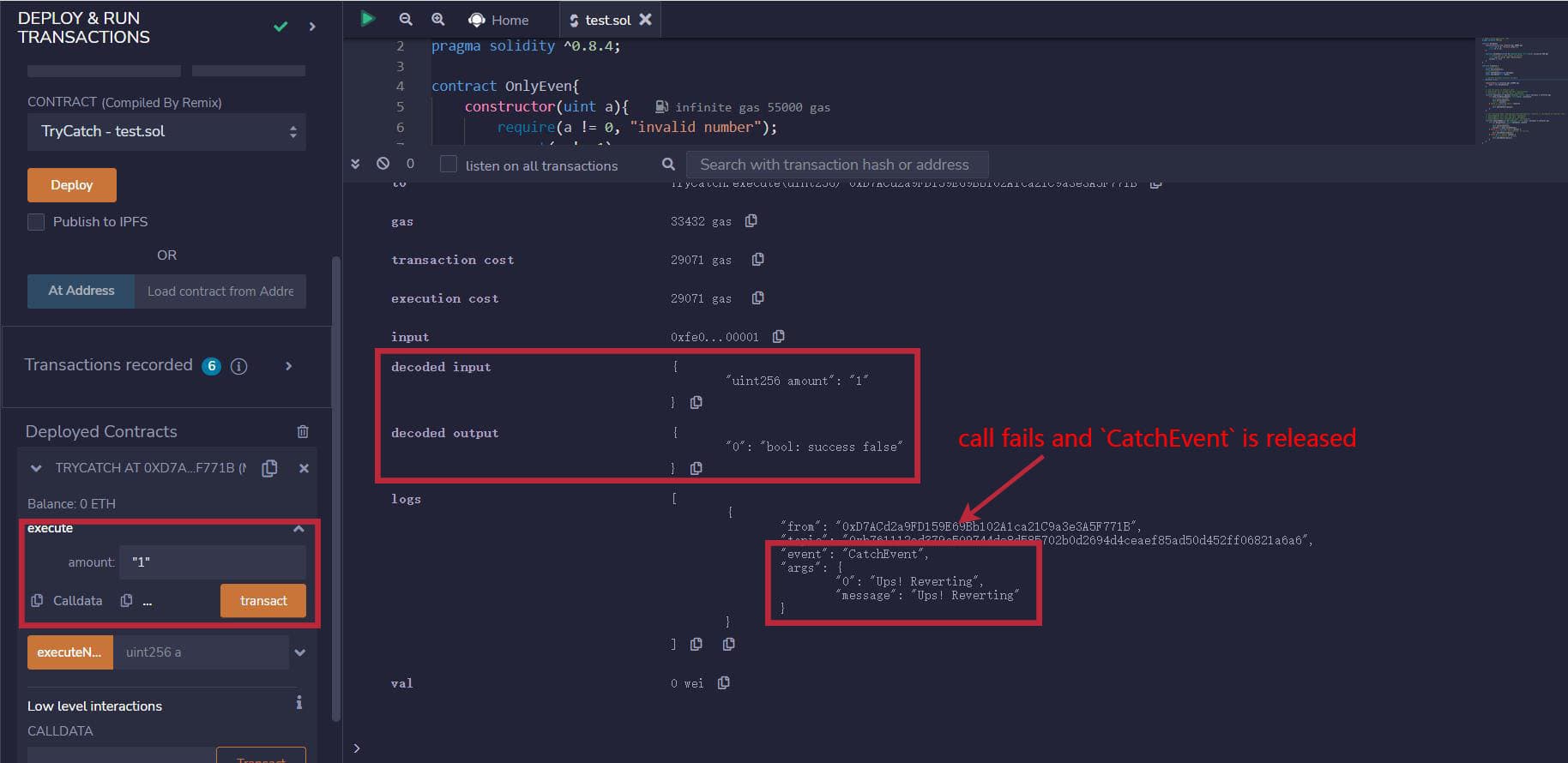
Handle contract creation exceptions
Here we use try-catch
to handle exceptions when a contract is created. Just need to rewrite try
module to the creation of OnlyEven
contract
verify on remix
When running executeNew(0)
, because 0
doesn't satisfy require(a != 0, "invalid number");
, the call will fail and CatchEvent
is released.
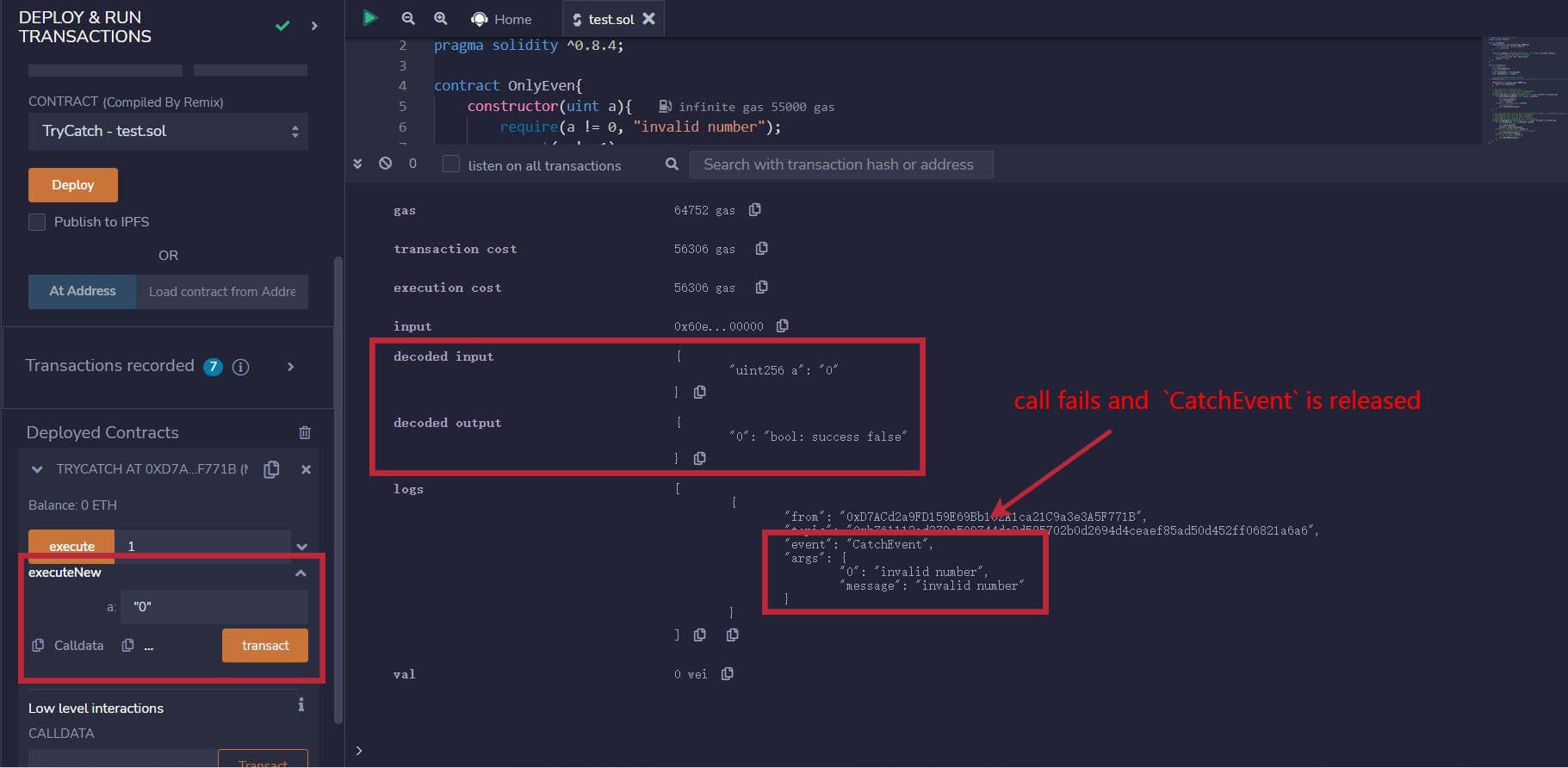
When running executeNew(1)
, because 1
doesn't satisfy assert(a != 1);
, the call will fail and CatchByte
is released.
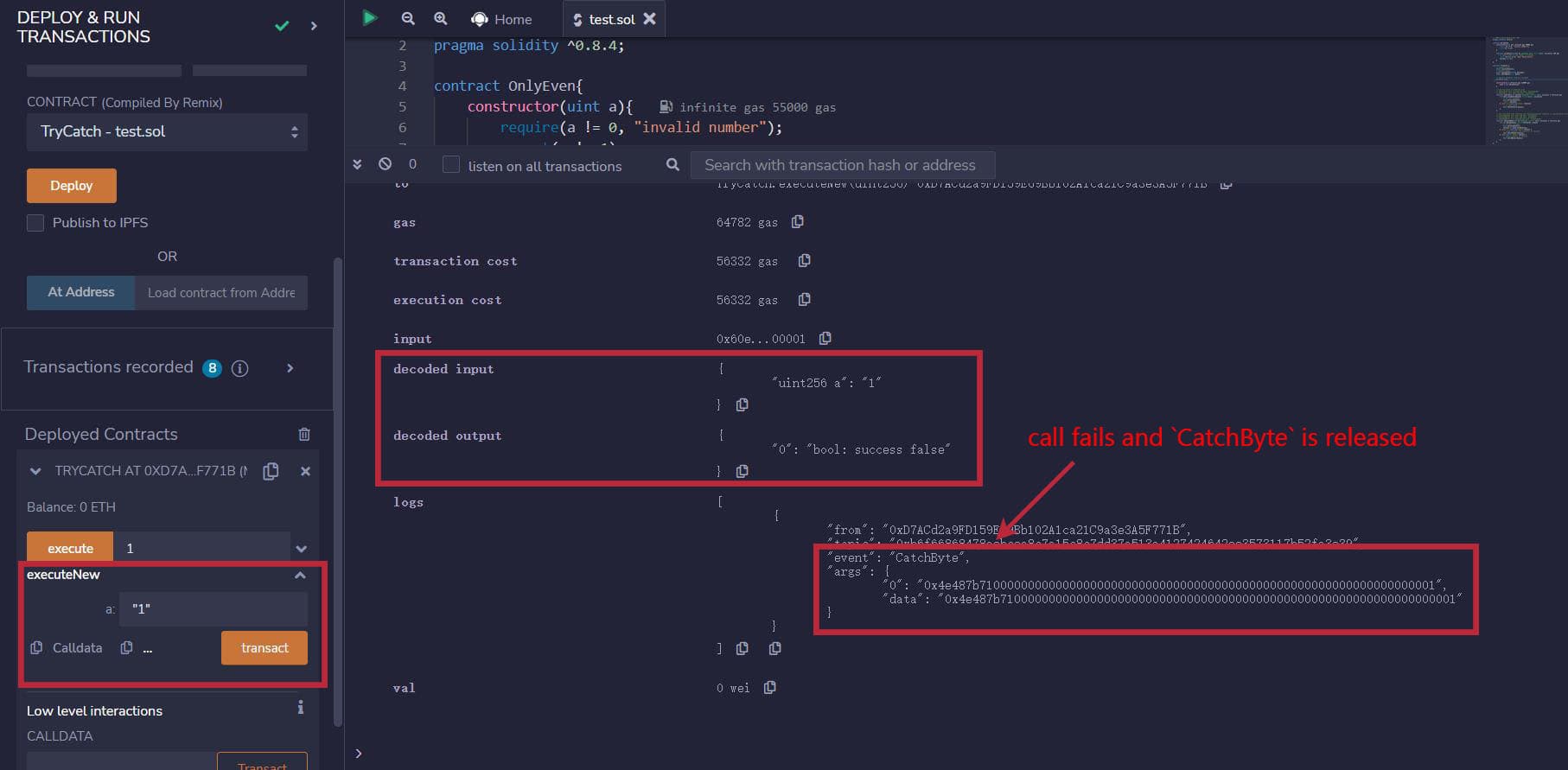
When running executeNew(2)
, because 2
satisfies require(a != 0, "invalid number");
and assert(a != 1);
, the call succeeds and SuccessEvent
is released.
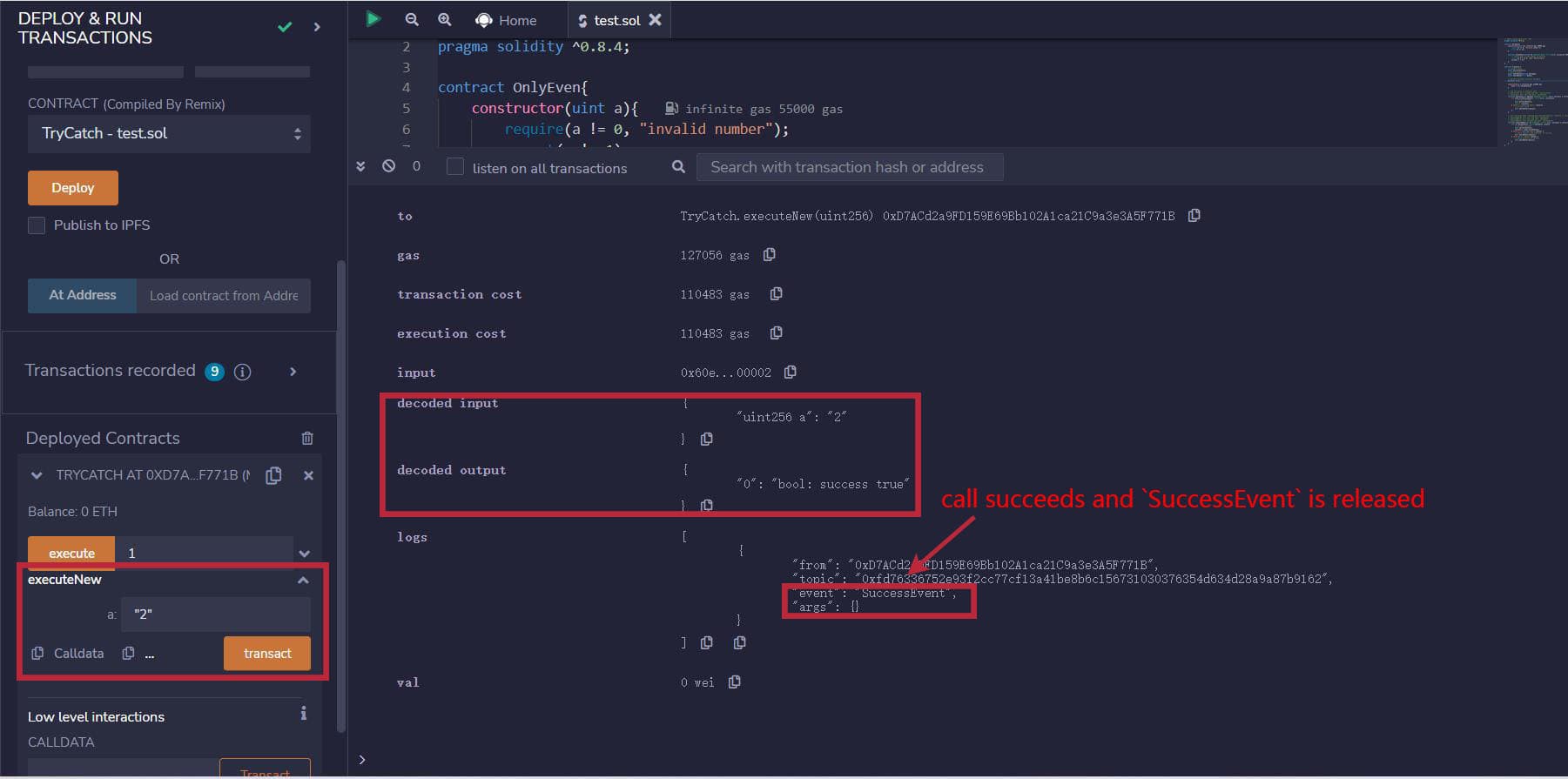
Summary
In this chapter, we introduced how to use try-catch
in solidity
to handle exceptions in the operation of smart contracts.