I've been revisiting ethers.js
recently to refresh my understanding of the details and to write a simple tutorial called "WTF Ethers" for beginners.
Twitter: @0xAA_Science
Community: Website wtf.academy | WTF Solidity | discord | WeChat Group Application
All the code and tutorials are open-sourced on GitHub: github.com/WTFAcademy/WTF-Ethers
In this lesson, we will introduce the Signer
class and its derived class Wallet
, and use it to send ETH
.
Signer
Class
Unlike Web3.js
, which assumes that users will deploy Ethereum nodes locally and manage private keys and network connection status through this node (which is not actually the case), ethers.js
manages network connection status with the Provider
class, and manages keys securely and flexibly with the Signer
class or Wallet
class, separately.
In ethers
, the Signer
class is an abstraction of an Ethereum account that can be used to sign messages and transactions, send signed transactions to the Ethereum network, and modify the blockchain state. The Signer
class is an abstract class and cannot be instantiated directly, so we need to use its subclass: the Wallet
class.
Wallet
Class
The Wallet
class inherits from the Signer
class. Developers can use it to sign transactions and messages just like they own an Externally Owned Account (EOA) with a private key.
Below are several ways to create instances of the Wallet
class:
- Creating a wallet object with a "known private key"
- Creating a wallet object with a "random private key"
- Creating a wallet object from a "mnemonic phrase / seed phrase"
- Creating a wallet object from a "JSON file"
For the sake of this tutorial, we will focus on number 1 above and briefly touch on others.
Method 1: Creating a wallet object with a known private key
If we know the private key, we can use the ethers.Wallet()
function to create a Wallet
object.
NB: For exercise purposes, connect a testnet address on your metamask with faucets.chain.link to get some usable ETH.
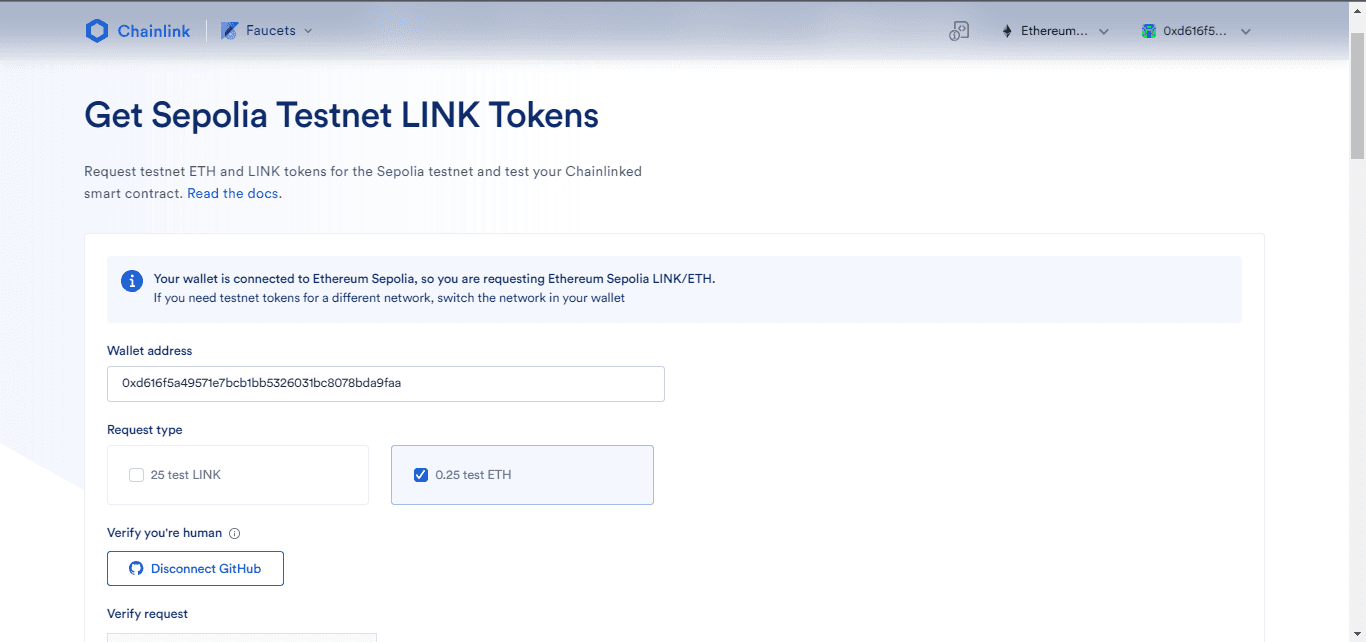
1. Create a Provider
instance
2. Create Wallet
instance
Create a Wallet
object with the private key for your testwallet. Wallets created using this method are standalone, and we need to use the connect(provider)
function to connect to the Ethereum node. Wallets created using this method can be used to obtain a mnemonic phrase.
3. Create an instance for both accounts before transfer and send ETH
4. Create an instance for both accounts after transfer and fetch balance using the getBalance()
function.
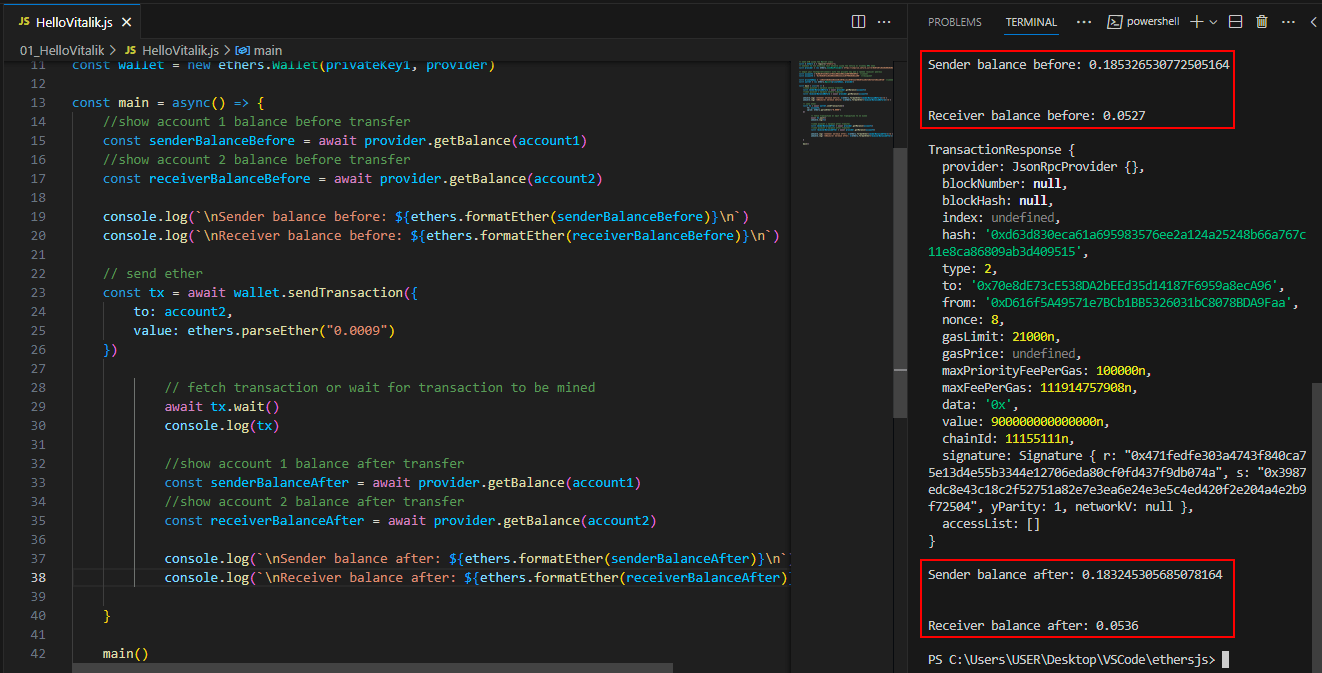
Other methods for creating a wallet object:
Method 2: Creating a random wallet object with a generated private key
We can use the ethers.Wallet.createRandom()
function to create a Wallet
object with a randomly generated private key. The private key is generated from an encrypted secure entropy source. If there is no secure entropy source in the current environment, an error will be thrown.
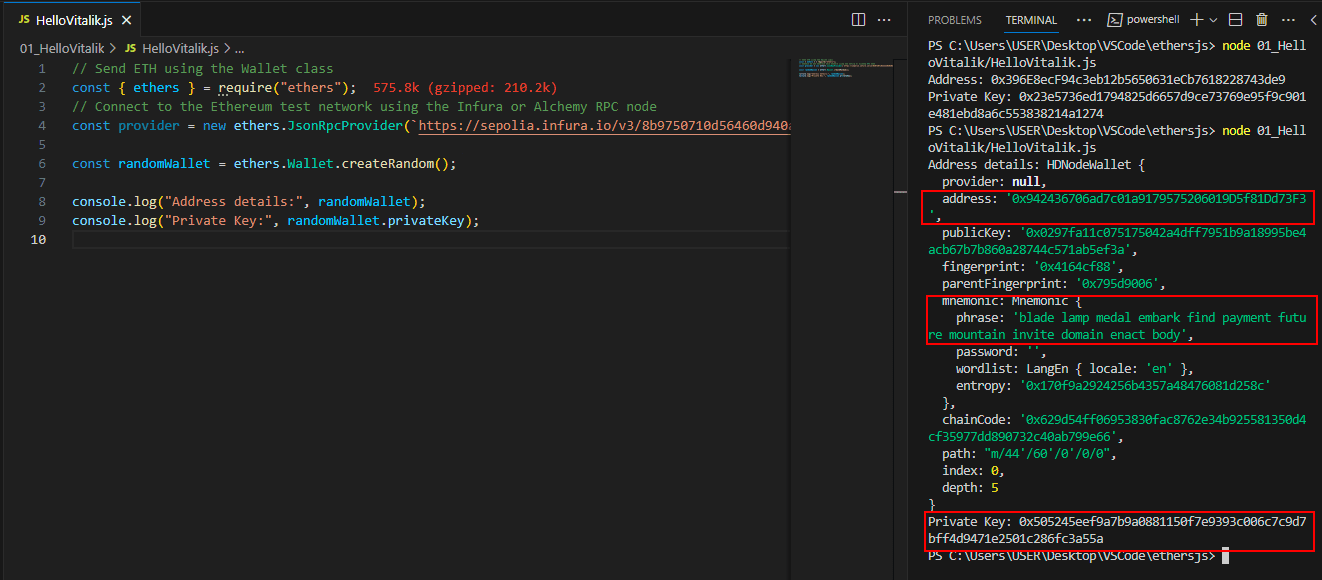
Method 3: Creating a wallet object from a mnemonic
If we know the wallet mnemonic/seed phrase, we can use the ethers.Wallet.fromMnemonic()
function to create a Wallet
object.
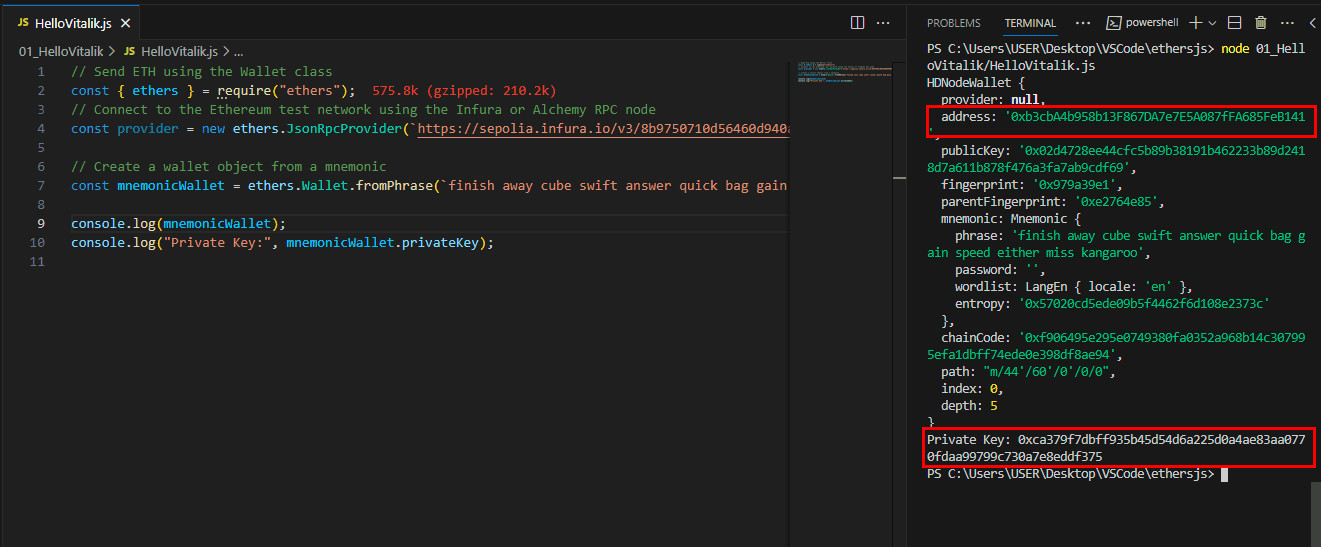
Method 4: Creating a wallet object from a JSON file
We can also create a wallet instance by decrypting a JSON wallet file using const wallet = ethers.Wallet.fromEncryptedJsonSync
decrypting a JSON
wallet file. A JSON
file is usually the keystore
file for wallets like Geth and Parity, which would be familiar to anyone who has set up an Ethereum node using Geth.
Summary
In this lesson, we introduced the Signer
and Wallet
classes and used the wallet instance to get the balance of a to
and from
addresses before and after the transaction. We also used some ethers.js functions to generate random wallets, mnemonic phrases, and private keys.